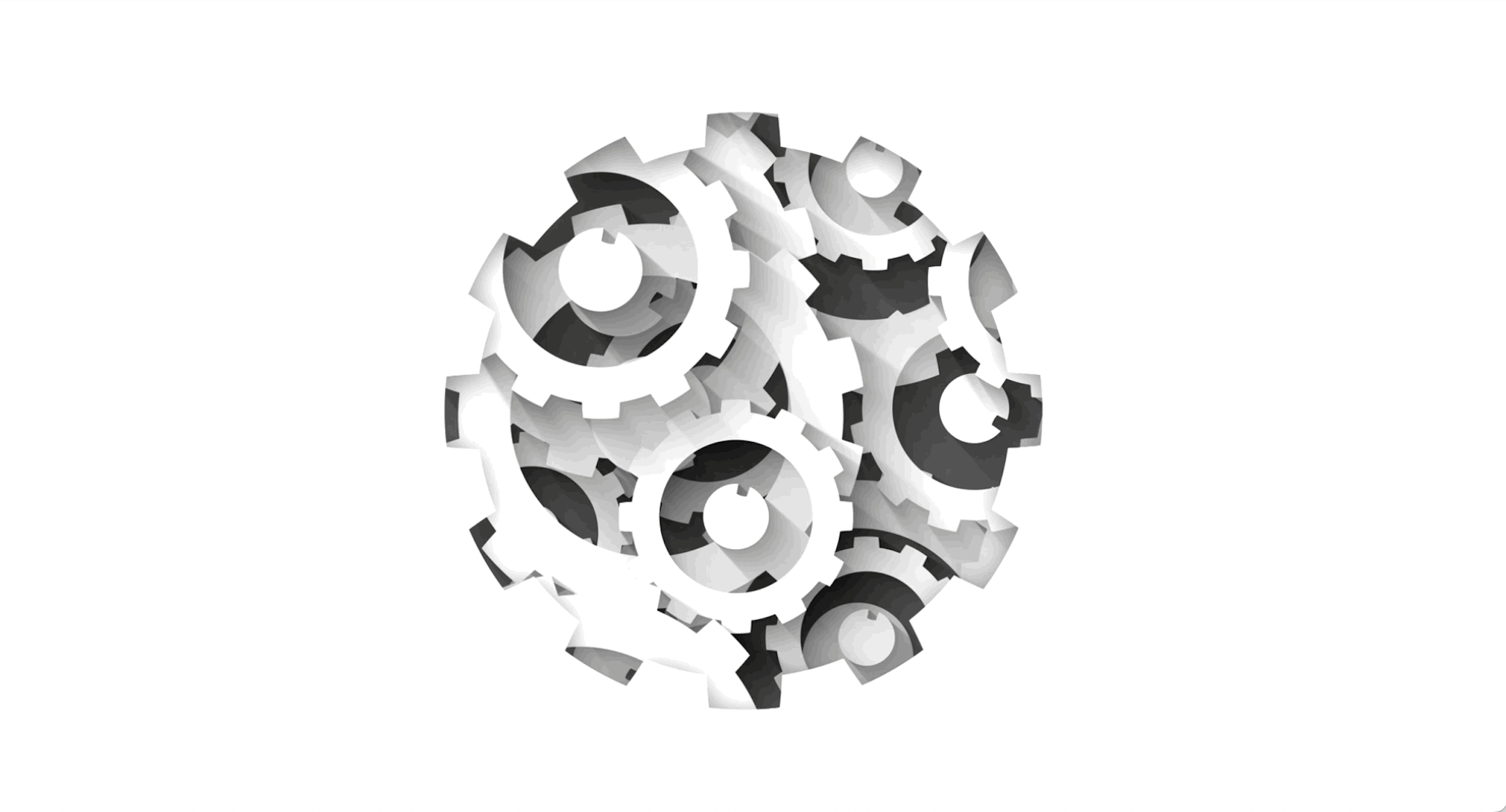
Test Observability & Engineering effectiveness
“Engineering Effectiveness” has been a central topic of discussion and focus this year
Testing across all interfaces, platforms and architectural components
Product test engineering, Shift-Left testing and digital transformation
Automate tests across all interfaces, platforms and horizontal scaling
Generative AI, Flutter, React Native, Micro-services, Micro-frontends & TestOps
Measure and enhance the efficiency & effectiveness of testing teams & solutions
Offshore Testing Setup as a Service, platform engineering and Modernisation
How to approach a complex-looking programming problem?
Solving programming problems can be complicated and overwhelming at first, but there are steps we can take to approach these complex-looking problems in a more manageable way.
The first step is to carefully read and understand the problem.
Make sure we identify –
There are a few existing techniques that have solved most, if not all, of the programming problems. The more such techniques you know the easier it will be to match and solve a given problem. All these techniques have a general format and only a few conditions change to solve different variations of the problems.
Below are some common techniques that you must have heard of-
Then there are a few others that are less known-
By practicing the techniques, we can approach even the most complex programming problems with confidence and come up with effective solutions.
For demonstration, let’s try to solve the following problem –
Question — Find the longest substring that has all non-repeating characters.
1. Input — A String (this can go as a parameter to our method)
String input = "aababcdefabcdefgbebe";
2. Output — A String — This is the longest substring from the given String that has all non-repeating characters in it
String output = "abcdefg";
3. Pattern — From our problem, we see that we need to find a substring (of variable length), which is continuous and that meets a given condition(non-repeating characters)
Sliding window technique — This technique works to find continuous SubString or SubArray from a given Sting/Array with the given condition.
In this given question, we need to find the longest SubString with a condition that characters should be non-repeating. This perfectly fits the definition of the sliding window technique.
Terminology
Solution Format
The code will probably look something like this –
while(end<length)
{
if() //condition where we get the required output and update the output variable
//for fixed size sliding window
else if() //condition where we can't get required output from out current window also condition where we move the window forward
//for variable size sliding window
else if() //condition where we can't get required output from out current window also the condition where we decrease window size by start++
}
Following is an article that lists some problems that could be solved using:
Sliding Window Technique
https://medium.com/techie-delight/top-problems-on-sliding-window-technique-8e63f1e2b1fa
That’s how we can solve most programming problems easily if we know the above-mentioned common programming techniques and the steps that solve them.
Do follow and subscribe to receive more of such articles.
Share This Article
“Engineering Effectiveness” has been a central topic of discussion and focus this year
With us, you’re not just a part of a company; you’re part of a movement dedicated to pushing boundaries, breaking barriers, and achieving the extraordinary.
Otaku 2.0 sought to redefine the way we approach testing, celebrate the spirit of innovation, and pave the way for a brighter future in tech.