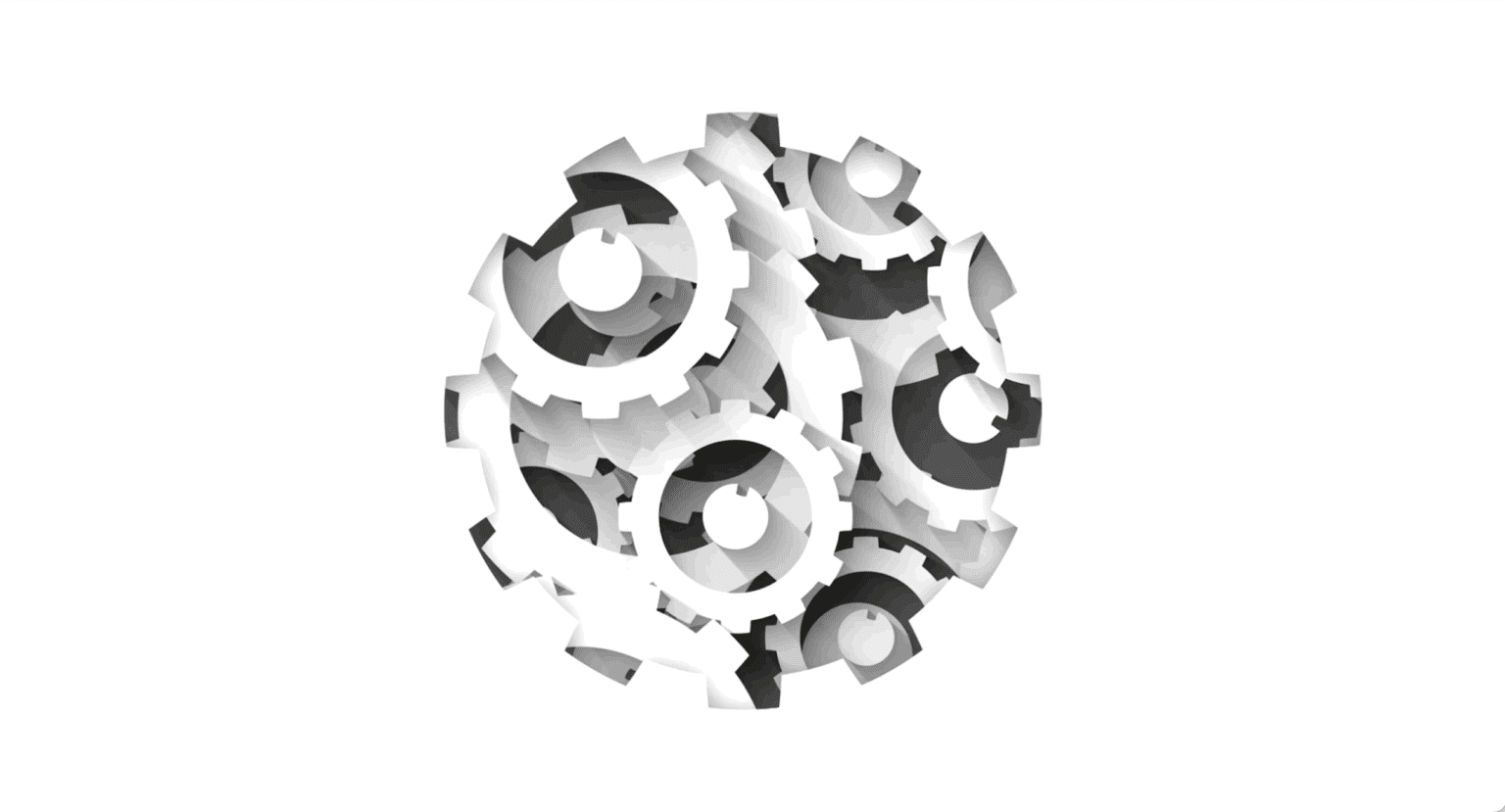
Test Observability & Engineering effectiveness
“Engineering Effectiveness” has been a central topic of discussion and focus this year
Testing across all interfaces, platforms and architectural components
Product test engineering, Shift-Left testing and digital transformation
Automate tests across all interfaces, platforms and horizontal scaling
Generative AI, Flutter, React Native, Micro-services, Micro-frontends & TestOps
Measure and enhance the efficiency & effectiveness of testing teams & solutions
Offshore Testing Setup as a Service, platform engineering and Modernisation
Best practices in JavaScript
JavaScript is a very forgiving language. It’s easy to write code that runs but has issues in it. In this article, we’ll look at some of the best practices in Java script.
The Factory Function is similar to constructor functions/class functions, but instead of using new to create an object, we can use factory functions to return objects in our way.
It is useful when inheritance is not required but we want to create objects.
And then we create object with
The object returns a function that doesn’t reference this
and it’s not an instance of any constructor.
Factory functions are reusable and can be composed.
If we want to add instance methods to a constructor function, we should add them to the prototype
property of the function.
For example, we can write:
Above code is better than adding a method inside the constructor as the property of this
as below
Duplication is not jus code that is exactly the same. Their implementation can also be duplicated.
Code with duplicate implementation can be extracted into a common location. Anything that violates the single responsibility principle has to be extracted into its own class.
We really don’t need two functions since the only difference between the two methods is the currency. Therefore we can combine them into one method and use the different currencies for different countries to calculate the total as follows.
Expressive code means easy to understand code. Our code has to communicate our intentions clearly so that the readers won’t misunderstand what we are trying to do.
Well-defined modules should be small and allow us to do a lot with little code. They don’t offer many functions to depend upon, so the coupling is loose.
A bad module has lots of functions that we have to call to get something done, so coupling is high.
Things that are exposed to modules should be minimized. Data and implementation should be hidden except when they can’t be. We shouldn’t create lots of instance variables that are inherited by other class.
Utility functions should be hidden from other modules. In the end, we should be able to reference a few things to do a lot to minimize coupling.
No more explanation 🙂
Anyone can write code that a computer can understand. Good programmers write code that humans can understand.
Share This Article
“Engineering Effectiveness” has been a central topic of discussion and focus this year
With us, you’re not just a part of a company; you’re part of a movement dedicated to pushing boundaries, breaking barriers, and achieving the extraordinary.
Otaku 2.0 sought to redefine the way we approach testing, celebrate the spirit of innovation, and pave the way for a brighter future in tech.