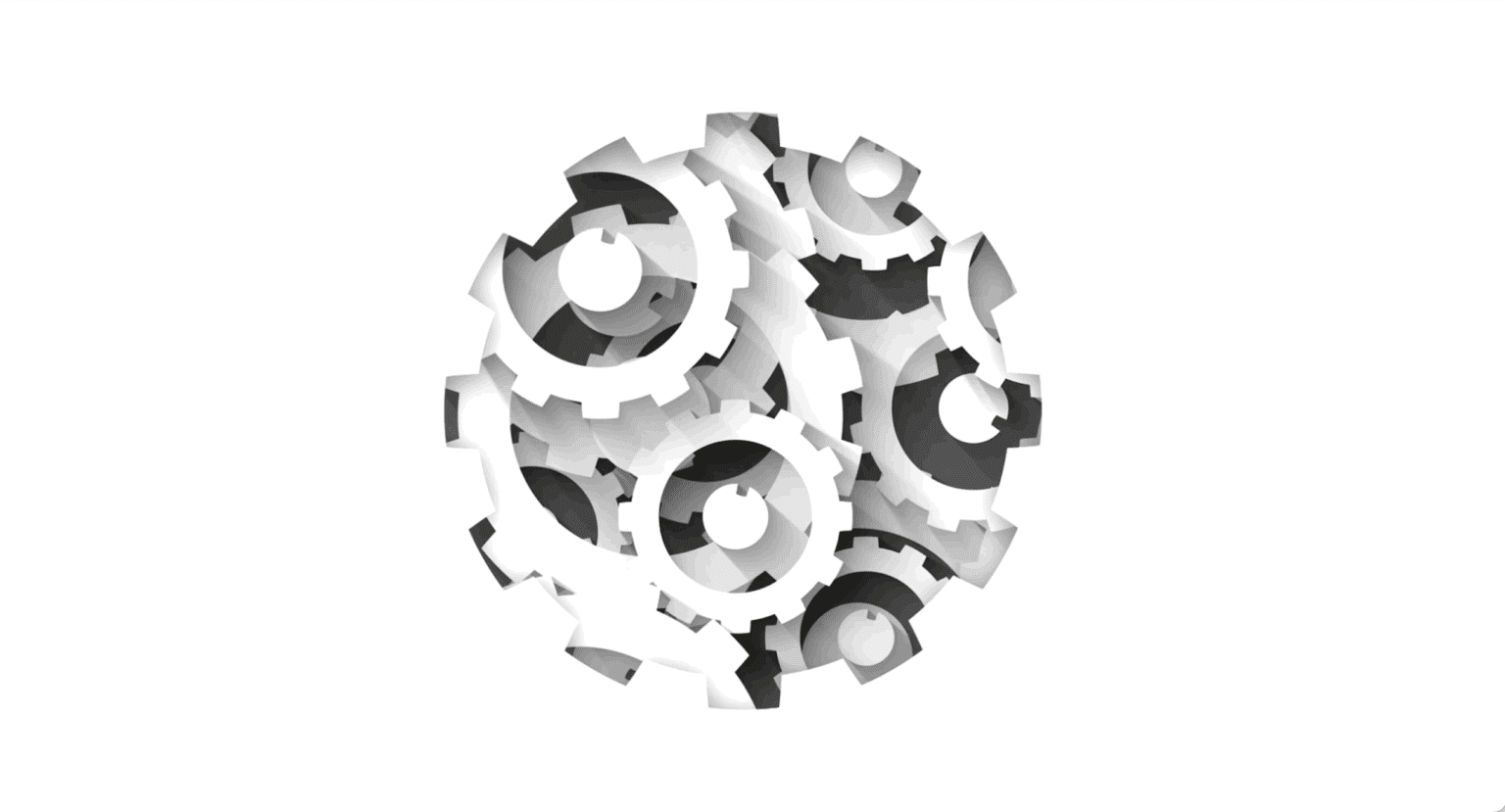
Test Observability & Engineering effectiveness
“Engineering Effectiveness” has been a central topic of discussion and focus this year
How to Automate gRPC tests?
gRPC is a modern open-source high-performance Remote Procedure Call (RPC) framework that can run in any environment. It can efficiently connect services in and across the data centers with pluggable support for load balancing, tracing, health checking, and authentication.
By default, gRPC uses Protocol Buffers (or Protobuf/protos), a binary encoding format developed by Google.
For more details like about .proto file, edge over REST, and gRPC service creation and deployment can be found given link.
Start by adding a dependency in our “build. gradle” file.
plugins {
id 'java'
id 'org.jetbrains.kotlin.jvm' version '1.3.72'
id "com.google.protobuf" version "0.8.8"
}
ext {
grpcVersion = "1.39.0"
protoVersion = "3.17.3"
}
dependencies {
testImplementation group: 'org.testng', name: 'testng', version: '7.4.0'
implementation "io.grpc:grpc-protobuf:${grpcVersion}"
implementation "io.grpc:grpc-stub:${grpcVersion}"
implementation "io.grpc:grpc-all:${grpcVersion}"
implementation group: 'javax.annotation', name: 'javax.annotation-api', version: '1.3.2'
runtimeOnly "io.grpc:grpc-netty-shaded:${grpcVersion}"
testImplementation "io.grpc:grpc-testing:${grpcVersion}"
}
“.proto” file will have all the API contracts, which means all requests, responses, and services names.
syntax = "proto3";
package bookstore;
option java_package = "com.endpoints.examples.bookstore";
option java_multiple_files = true;
option java_outer_classname = "BookstoreProto";
service BookService {
rpc GetBook (GetBookRequest) returns (BookResponse) {}
rpc GetBooksViaAuthor (BookAuthorRequest) returns (BookResponse) {}
}
message BookResponse {
string message = 1 ;
string response_code = 2;
}
message GetBookRequest {
int32 isbn = 1;
}
message BookAuthorRequest {
string author = 1;
}
“com. google. protobuf” plugin is used to create stubs in the desired language.
Run
./gradlew clean build
We are creating a Java Client which will be interacting with gRPC services. Our Test code will interact with services via client only.
public class BookClient {
ManagedChannel channel;
BookServiceGrpc.BookServiceBlockingStub bookServiceStub;
public BookClient() {
channel = ManagedChannelBuilder.forAddress("localhost", 50055).usePlaintext().build();
bookServiceStub = BookServiceGrpc.newBlockingStub(channel);
}
public BookResponse getBookByISBN(Integer ISBN) throws BookNotFoundException {
try {
GetBookRequest getBookRequest = GetBookRequest.newBuilder().setIsbn(ISBN).build();
return bookServiceStub.getBook(getBookRequest);
} catch (Exception ex) {
System.out.println(ex.getMessage());
throw new BookNotFoundException("Book not found");
}
}
}
Finally, writing out test code for the gRPC service.
public class BookTests extends BaseTests {
private BookClient bookClient;
public BookTests() {
bookClient = new BookClient();
}
@Test
public void getBookTests() throws BookNotFoundException {
BookResponse bookResponse = bookClient.getBookByISBN(1);
Assert.assertEquals(bookResponse.getResponseCode(), "200");
}
@Test
public void getBookViaAuthor() throws AuthorNotFoundException {
BookResponse bookResponse = bookClient.getBookByAuthor("Bob");
Assert.assertEquals(bookResponse.getResponseCode(), "200");
}
}
We can use BloomRPC as an alternative to test gRPC services.BloomRPC is a GUI tool, to test gRPC services likewise Postman for REST.
Share This Article
“Engineering Effectiveness” has been a central topic of discussion and focus this year
With us, you’re not just a part of a company; you’re part of a movement dedicated to pushing boundaries, breaking barriers, and achieving the extraordinary.
Otaku 2.0 sought to redefine the way we approach testing, celebrate the spirit of innovation, and pave the way for a brighter future in tech.